API Access
The ACC provides access to basic functionality through an API. The following API calls are available:
Authentication
The API requires authentication which must be configured in the ACC Web Portal. You must add an access token to a user account in the ACC, and then use this access token in all API calls. We recommend you to create a dedicated user account for API access. You cannot add an access key to a Super Admin user.
Follow these below steps to create an API access token. Only administrators can create access tokens:
Open the Users page and select API Access in the context menu for the user you want to give API access.
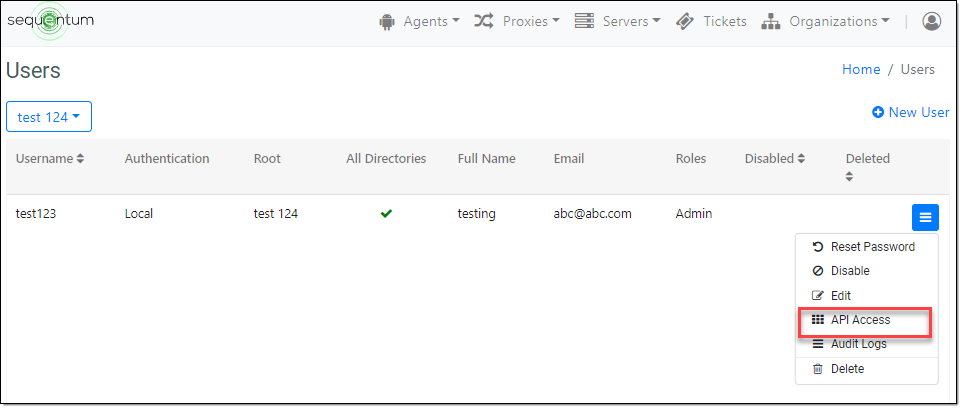
2. Click Add Access Token and create a new token. A date can be specified as to when the Access Token will expire.
3. Copy the access token from the list or select Copy to Clipboard from the context menu.
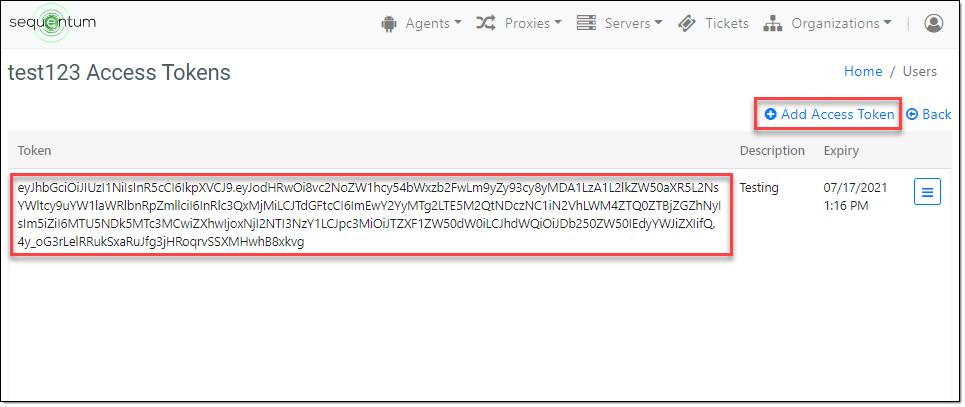
The authentication token must be added to every API request headers. The following request shows how the access token is added to the Authorization header.
GET /api/agent?agentPath=sequentum/Test2/quick HTTP/1.1
Host: acc.sequentum.com
Authorization: Bearer
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6IlJvb3QiLCJTdGFtcCI6ImNjOWNiN2JhLWM0ZjItNGM0NS05ODY4LTMxM2Y5ZDRmZjhkZiIsIm5iZiI6MTU1ODQwNTI4NiwiZXhwIjoxNTkwMDI3NjgxLCJpc3MiOiJTZXF1ZW50dW0iLCJhdWQiOiJDb250ZW50IEdyYWJiZXIifQ.2pFQY9Ag8jndcL4jBSVLm_rhsZpmXXS8nn_crqXZ85c
Accept:*/*
Cache-Control: no-cache
accept-encoding: gzip, deflate
Connection: keep-alive
Get agent details from repository
GET api/agent?agentPath={agentPath}
Returns information about an agent's Id, name, directory Id, and description in the ACC Repository. The agentPath is a full path to an agent.
This API call is often the first call you'll make, so you can get the Agent ID which is used in many of the other API calls.
Example:
API Request
Python API RequestPython APPython API RequestI Request
import requests
url = "https://acc.sequentum.com/api/agent?agentPath=sequentum/Test2/quick"
payload = {}headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6IlJvb3QiLCJTdGFtcCI6ImNjOWNiN2JhLWM0ZjItNGM0NS05ODY4LTMxM2Y5ZDRmZjhkZiIsIm5iZiI6MTU1ODQwNTI4NiwiZXhwIjoxNTkwMDI3NjgxLCJpc3MiOiJTZXF1ZW50dW0iLCJhdWQiOiJDb250ZW50IEdyYWJiZXIifQ.2pFQY9Ag8jndcL4jBSVLm_rhsZpmXXS8nn_crqXZ85c'}
response = requests.request("GET", url, headers=headers, data = payload)print(response.text.encode('utf8'))
Output:
{
"agentId": "a2f22850-7c88-4e05-a30a-004aa980ee6c",
"name": "quick",
"directoryId": "45e52cf1-b2a1-4f37-b670-4582c08a8df8",
"description": null
}
Start agent on cluster
POST api/agent/{agentId}/cluster/start
or
POST api/agent/cluster/start?agentPath=agentPath
Body: {"sessionId":"sessionId", "logLevel": 0|1|2|3, "isProductionRun": true|false, "inputParameters": "{\"name1\":\"value1\",\"name2\":\"value2\"}", "inputFiles": ["input1.csv", "input2.csv"], "isDeleteInputFilesOnCompleted": false, "useMultipleSessions": true}
Starts an agent run on the cluster where the agent is deployed.
agentId - The ID of the agent to start.
agentPath - The path to the agent in the Agent Repository. For example: sequentum/Test1/Test-Agent.
sessionId (optional - default value is empty) - The session ID to start. The agent must support sessions.
logLevel (optional - default value is 1) - The log detail level. 0 means no logging and 3 is the highest detail level.
isProductionRun (optional - default value is true) - Data delivery and email notifications are turned off for non-production runs.
inputParameters (optional - default value is an empty list) - name/value pairs to use as agent input parameters.
inputFiles (optional - default value is an empty list) - Input files to use for this run. The input files must have been previously uploaded. Input files are provided to the agent as input parameters with the names "input_file_1", "input_file_2", ...
Note: The supported input file formats are .csv and .txt and also the agent needs to be configured with the recommended changes below so that the agent can accept any newly uploaded files and use the newly uploaded file inputs in the agent data provider command.
When an input CSV/txt file is used, add an Input Parameter named "input_file_1" with a path to the input file. Then, on the data provider command, use [input_file_1] as the CSV input file path. The square brackets instruct the data provider to look for the file path in the specified input parameter.
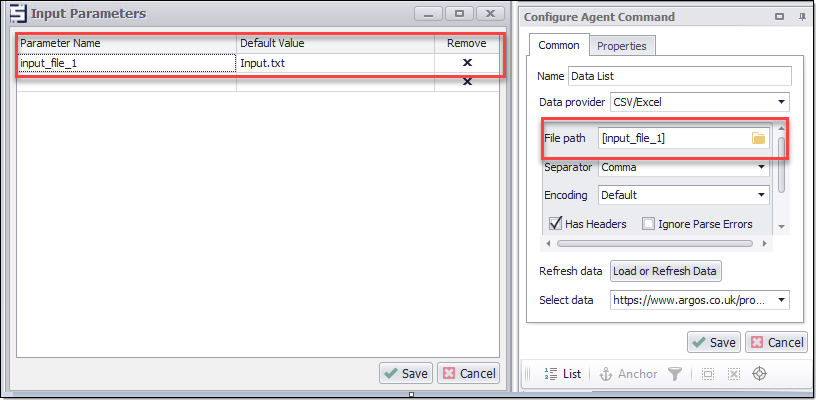
isDeleteInputFilesOnCompleted (optional - default value is false) - Deletes the specified input files when the agent run has completed successfully or has been manually set to failed.
useMultipleSessions (optional - default value is false) - Automatically starts new sessions, allowing multiple instances of the same agent to run simultaneously. The sessionId must be empty, and the agent must be configured to support multiple sessions. The agent should be configured to remove sessions immediately on success and session timeout should be set to a long period of time such as 10800 minutes (one week). A value of 10800 minutes will give you one week to rerun or retry a failed agent run before the session is deleted.
isSynchronous (optional - default value is false) - If true, the call will not return before the run has completed.
synchronousTimeout (optional - default value is 60) - The maximum number of seconds an agent is allowed to run synchronously before the agent is stopped and the call returns. The default value is 60.
synchronousDataDelivery (optional - default value is None) - Specifies if this call returns extracted data when run asynchronously. The value can be None, Plain or Zip. The agent run IDs will be returned as normal when this option is set to None. Plain text data will be returned when the option is set to Plain. When returning plain text, only data from the first data file will be returned when an agent exports more than one data file. A ZIP file with ALL data files will be returned when this option is set to Zip.
Example:
API Request
Python API RequestP
POST https://acc.sequentum.com/api/agent/a2f22850-7c88-4e05-a30a-004aa/cluster/start
Body: {
"logLevel":1,
"isProductionRun": true,
"inputParameters": "{\"input1\":\"my input 1\",\"input2\":\"my input 2\"}"
}
import requests
url = "https://acc.sequentum.com/api/agent/a2f22850-7c88-4e05-a30a-004aa/cluster/start"payload = "{\"logLevel\": 1,\"isProductionRun\": true,\"inputParameters\":\"{\\\"input1\\\":\\\"my input 1\\\",\\\"input2\\\":\\\"my input 2\\\"}\"}"
headers = {'Content-Type': 'application/json','Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6IlJvb3QiLCJTdGFtcCI6ImNjOWNiN2JhLWM0ZjItNGM0NS05ODY4LTMxM2Y5ZDRmZjhkZiIsIm5iZiI6MTU1ODQwNTI4NiwiZXhwIjoxNTkwMDI3NjgxLCJpc3MiOiJTZXF1ZW50dW0iLCJhdWQiOiJDb250ZW50IEdyYWJiZXIifQ.2pFQY9Ag8jndcL4jBSVLm_rhsZpmXXS8nn_crqXZ85c'}
response = requests.request("POST", url, headers=headers, data = payload)print(response.text.encode('utf8'))
Output:
{"agentRunHistoryIds": ["a2f6782850-7c89-4e05-a30a-034aa980ee6c"]}
agentRunHistoryIds - A list of IDs that identify the agent runs that have started. The list will always consist of a single ID unless performance sessions are used.
The call may return extracted data instead of the run IDs if the option synchroneousDataDelivery is set to Plain or Zip.
Restart run
POST api/run/{agentRunHistoryId}/restart
Restarts a specific agent run.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/run/9040f86d-bbc1-4038-9868-501145cc2370/restart"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)print(response.text.encode('utf8'))
Retry run
POST api/run/{agentRunHistoryId}/retry?RunMethod=ContinueAndRetryErrors
Retries errors for a specific agent run. This method requires a RunMethod query parameter set to one of these below:
Restart
Continue
ContinueRefreshAgent
ContinueAndRetryErrors
ContinueAndRetryErrorsRefreshAgent
New
The default value is ContinueAndRetryErrorsRefreshAgent.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/run/e93d698f-2bca-c6b4-0ac9-39f5e9f71653/retry?RunMethod=ContinueAndRetryErrorsRefreshAgent"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)print(response.text.encode('utf8'))
Get agent run details
GET api/run/{agentRunHistoryId}
Returns information about the specified agent run.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/run/9040f86d-bbc1-4038-9868-501145cc2370"
payload = {}
headers = {'Authorization': 'Bearer
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Output:
{
"agentRunHistoryId": "9040f86d-bbc1-4038-9868-501145cc2370",
"agentId": "a2f22850-7c88-4e05-a30a-004aa980ee6c",
"sessionId": null,
"runId": 0,
"agentJobScheduleId": null,
"serverIp": "127.0.0.1",
"startTime": "2019-05-21T13:52:14.3020127+10:00",
"endTime": "2019-05-21T13:52:23.6568249+10:00",
"expectedEndTime": null,
"runTime": "00:00:08.9677836",
"status": 4,
"successActionCount": 1,
"dynamicSuccessActionCount": 1,
"volume": 25,
"pageLoadCount": 1,
"requestCount": 33,
"downloadCount": 0,
"errors": 0,
"dataCount": 1,
"deliveryCount": 0,
"statusMessage": "Completed",
"logPath": "quick-2019_05_21-01_52_14.log",
"inputParameters": null,
"logLevel": 1,
"isWindowLess": true,
"isProductionRun": false,
"backupDataExpiry": null,
"lastUpdated": "2019-05-21T13:52:23.656843+10:00"
}
Run status can be one of the following values:
0 - Never Run
1 - Starting
2 - Running
3 - Delivery
4 - Succeeded
5 - Failed
6 - Stopped
7 - Failure
Get agent run history
GET api/agent/{agentId}/history?count={count}
Returns information about the latest agent runs. Run history includes information about active agent runs. The latest 100 agent runs are returned if the count is not specified.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/agent/a2f22850-7c88-4e05-a30a-004aa980ee6c/history?count=1"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Output:
[
{
"agentRunHistoryId": "9040f86d-bbc1-4038-9868-501145cc2370",
"agentId": "a2f22850-7c88-4e05-a30a-004aa980ee6c",
"sessionId": null,
"runId": 0,
"agentJobScheduleId": null,
"serverIp": "127.0.0.1",
"startTime": "2019-05-21T13:52:14.3020127+10:00",
"endTime": "2019-05-21T13:52:23.6568249+10:00",
"expectedEndTime": null,
"runTime": "00:00:08.9677836",
"status": 4,
"successActionCount": 1,
"dynamicSuccessActionCount": 1,
"volume": 25,
"pageLoadCount": 1,
"requestCount": 33,
"downloadCount": 0,
"errors": 0,
"dataCount": 1,
"deliveryCount": 0,
"statusMessage": "Completed",
"logPath": "quick-2019_05_21-01_52_14.log",
"inputParameters": null,
"logLevel": 1,
"isWindowLess": true,
"isProductionRun": false,
"backupDataExpiry": null,
"lastUpdated": "2019-05-21T13:52:23.656843+10:00"
}
]
Run status can be one of the following values:
0 - Never Run
1 - Starting
2 - Running
3 - Delivery
4 - Succeeded
5 - Failed
6 - Stopped
7 - Failure
Start Job
POST api/job/{agentId}/start
Body: {"sessionId":"sessionId", "logLevel": 0|1|2|3, "isProductionRun": true|false, "inputParameters": "{\"name1\":\"value1\",\"name2\":\"value2\"}", "inputFiles": ["input1.csv", "input2.csv"], "isDeleteInputFilesOnCompleted": true|false}
Starts an agent job run. A job keeps track of all runs started by the job, so it's often used together with performance sessions. Multiple job for the same agent cannot run simultaneously.
agentId - The ID of the agent to start.
sessionId (optional - default value is empty) - The session ID to start. The agent must support sessions.
logLevel (optional - default value is 1) - The log detail level. 0 means no logging and 3 is the highest detail level.
isProductionRun (optional - default value is true) - Data delivery and email notifications are turned off for non-production runs.
inputParameters (optional - default value is an empty list) - name/value pairs to use as agent input parameters.
inputFiles (optional - default value is an empty list) - Input files to use for this run. The input files must have been previously uploaded. Input files are provided to the agent as input parameters with the names "input_file_1", "input_file_2", ...
Note: The supported input file formats are .csv and .txt and also the agent needs to be configured with the recommended changes below so that the agent can accept any newly uploaded files and use the newly uploaded file inputs in the agent data provider command.
When an input CSV/txt file is used, add an Input Parameter named "input_file_1" with a path to the input file. Then, on the data provider command, use [input_file_1] as the CSV input file path. The square brackets instruct the data provider to look for the file path in the specified input parameter.
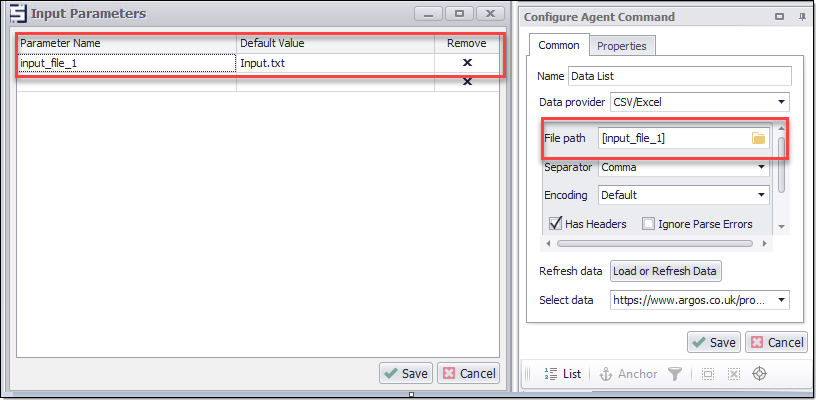
isDeleteInputFilesOnCompleted (optional - default value is false) - Deletes the specified input files when the job run has completed successfully or has been manually set to failed.
Example:
API Request
Python API RequestP
POST https://acc.sequentum.com/api/job/a2f22850-7c88-4e05-a30a-004aa980ee6c/start
Body: {
"logLevel":1,
"isProductionRun": true,
"inputParameters": "{\"input1\":\"my input 1\",\"input2\":\"my input 2\"}"
}
import requests
url = "https://acc.sequentum.com/api/job/a2f22850-7c88-4e05-a30a-004aa980ee6c/start"
payload = "{\"logLevel\": 1,\"isProductionRun\": true,\"inputParameters\":\"{\\\"input1\\\":\\\"my input 1\\\",\\\"input2\\\":\\\"my input 2\\\"}\"}"
headers = {'Content-Type': 'application/json',
'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Output:
{"agentJobHistoryId": "a2f6782850-7c89-4e05-a30a-034aa980ee6c", "jobRunId":2}
agentJobHistoryId - An ID that identifies the job run.
jobRunId - An increasing number that identifies the job run for a specific agent.
Get job run details
Returns information about the specified job run.
GET api/job/run/{agentJobHistoryId}
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/run/a2f22850-7c88-4e05-a30a-004aa980ee6c"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Output:
{
"agentJobHistoryId": "6ef84ec7-d5e6-4a98-bbfa-0b67355a648f",
"agentId": "a2f22850-7c88-4e05-a30a-004aa980ee6c",
"startTime": "2019-05-19T15:55:47.4225218+10:00",
"endTime": "2019-05-19T15:55:58.9723817+10:00",
"totalSuccessActionCount": 1,
"totalDynamicSuccessActionCount": 1,
"totalPageLoadCount": 1,
"totalRequestCount": 33,
"totalDownloadCount": 0,
"totalVolume": 25,
"totalErrors": 0,
"totalDataCount": 1,
"totalDeliveryCount": 1,
"completedScheduleCount": 1,
"totalScheduleCount": 1,
"completedRunCount": 1,
"totalRunCount": 1,
"runId": 3,
"status": 3,
"agentJobSetting": null
}
The job status can be one of the following values:
0 - Never Run
1 - In Progress
2 - In Progress With Failure
3 - Succeeded
4 - Failure
5 - Failed
Get job run history
Returns information about the specified number of latest job runs. Job history includes information about active job runs. The latest 100 job runs are returned if the count is not specified.
GET api/job/{agentId}/history?count=1
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/a2f22850-7c88-4e05-a30a-004aa980ee6c/history?count=2"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Output:
[
{
"agentJobHistoryId": "6ef84ec7-d5e6-4a98-bbfa-0b67355a648f",
"agentId": "a2f22850-7c88-4e05-a30a-004aa980ee6c",
"startTime": "2019-05-19T15:55:47.4225218+10:00",
"endTime": "2019-05-19T15:55:58.9723817+10:00",
"totalSuccessActionCount": 1,
"totalDynamicSuccessActionCount": 1,
"totalPageLoadCount": 1,
"totalRequestCount": 33,
"totalDownloadCount": 0,
"totalVolume": 25,
"totalErrors": 0,
"totalDataCount": 1,
"totalDeliveryCount": 1,
"completedScheduleCount": 1,
"totalScheduleCount": 1,
"completedRunCount": 1,
"totalRunCount": 1,
"runId": 3,
"status": 3,
"agentJobSetting": null
},
{
"agentJobHistoryId": "156aba0d-bb4f-4244-96ef-73d89aaa47ec",
"agentId": "a2f22850-7c88-4e05-a30a-004aa980ee6c",
"startTime": "2019-05-16T17:30:17.6996551+10:00",
"endTime": "2019-05-16T17:56:49.4434358+10:00",
"totalSuccessActionCount": 1,
"totalDynamicSuccessActionCount": 1,
"totalPageLoadCount": 1,
"totalRequestCount": 31,
"totalDownloadCount": 0,
"totalVolume": 25,
"totalErrors": 0,
"totalDataCount": 0,
"totalDeliveryCount": 1,
"completedScheduleCount": 1,
"totalScheduleCount": 1,
"completedRunCount": 1,
"totalRunCount": 1,
"runId": 2,
"status": 3,
"agentJobSetting": null
}
]
The job status can be one of the following values:
0 - Never Run
1 - In Progress
2 - In Progress With Failure
3 - Succeeded
4 - Failure
5 - Failed
Get agent run history for a job run
GET api/agent/{agentId}/run/{runId}/history
Returns information about all agent runs associated with a specific job run.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/agent/a2f22850-7c88-4e05-a30a-004aa980ee6c/run/5/history?count=1"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Output:
[
{
"agentRunHistoryId": "9040f86d-bbc1-4038-9868-501145cc2370",
"agentId": "a2f22850-7c88-4e05-a30a-004aa980ee6c",
"sessionId": null,
"runId": 0,
"agentJobScheduleId": null,
"serverIp": "127.0.0.1",
"startTime": "2019-05-21T13:52:14.3020127+10:00",
"endTime": "2019-05-21T13:52:23.6568249+10:00",
"expectedEndTime": null,
"runTime": "00:00:08.9677836",
"status": 4,
"successActionCount": 1,
"dynamicSuccessActionCount": 1,
"volume": 25,
"pageLoadCount": 1,
"requestCount": 33,
"downloadCount": 0,
"errors": 0,
"dataCount": 1,
"deliveryCount": 0,
"statusMessage": "Completed",
"logPath": "quick-2019_05_21-01_52_14.log",
"inputParameters": null,
"logLevel": 1,
"isWindowLess": true,
"isProductionRun": false,
"backupDataExpiry": null,
"lastUpdated": "2019-05-21T13:52:23.656843+10:00"
}
]
Run status can be one of the following values:
0 - Never Run
1 - Starting
2 - Running
3 - Delivery
4 - Succeeded
5 - Failed
6 - Stopped
Get job schedules
GET api/job/{agentId}/schedules
Returns information about all schedules associated with a Job.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/a2f22850-7c88-4e05-a30a-004aa980ee6c/schedules"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Output:
[
{
"scheduleId": "d9c944df-93a7-ea77-f7b7-fa0b9c579917",
"agentId": "a2f22850-7c88-4e05-a30a-004aa980ee6c",
"cron": "0 0 0 1",
"cronDescription": "Runs every day at 3:36:00 PM (AET).",
"sessionId": null,
"startTime": "2019-05-30T15:36:00",
"timeZoneId": "AUS Eastern Standard Time",
"nextRunTime": "2019-05-30T15:36:00+10:00",
"inputParameters": "{}",
"logLevel": 2,
"isWindowless": true,
"errorMessage": null,
"scheduleStatus": 2,
"lastRunTime": "2019-05-21T15:26:42.967476+10:00",
"completedTime": "2019-05-21T15:26:52.6831583+10:00",
"isEnabled": true
}
]
Schedule status can be one of the following values:
0 - Waiting
1 - Running
2 - Succeeded
3 - Failed
Add or update job schedule
POST /api/job/{agentId}/schedule
Body:
{"scheduleId":"b4cdc56a-2c16-4b1b-a135-b4e687f8bc95",
"cron":"0 0 0 1","sessionId":null,
"startTime":"2019-07-16T11:21:20","timeZoneId":"AUS Eastern Standard Time",
"inputParameters":"{\"par1\":\"2000\",\"par2\":\"5000\"}",
"logLevel":1,"isWindowless":true,"isEnabled":true}
Adds a new job schedule to a specified agent or updates an existing job schedule.
agentId - The ID of the agent.
scheduleId - The ID of the schedule to add.
cron - The Cron expression which specifies when and how often to run the the schedule. Please read Cron Expressions for more information about Cron syntax.
startTime - The start date and time of the schedule. Must be in the following format: yyyy-MM-ddTHH:mm:ss
timeZoneId - The time zone of the schedule. Read List of Time Zones for more details.
sessionId (optional - default value is empty) - The session ID. The agent must support sessions.
logLevel (optional - default value is 1) - The log detail level. 0 means no logging and 3 is the highest detail level.
inputParameters (optional - default value is an empty list) - name/value pairs to use as agent input parameters.
isWindowless - Specifies whether the schedule will run windowless or not.
isEnabled - Specifies whether the schedule is enabled or not.
Example:
API Request
Python API RequestP
POST https://acc.sequentum.com/api/job/0041d691-49d1-4c48-8c21-654b8e248c11/schedule
Body: {
"scheduleId":"b4cdc56a-2c16-4b1b-a135-b4e687f8bc95",
"cron":"0 0 0 1",
"sessionId":null,
"startTime":"2019-07-16T11:21:20",
"timeZoneId":"AUS Eastern Standard Time",
"inputParameters":"{\"par1\":\"2000\",\"par2\":\"5000\"}",
"logLevel":1,
"isWindowless":true,
"isEnabled":true
}
import requests
url = "https://acc.sequentum.com/api/job/0041d691-49d1-4c48-8c21-654b8e248c11/schedule"
payload = "{\"scheduleId\":\"b4cdc56a-2c16-4b1b-a135-b4e687f8bc95\",\"cron\":\"0 0 0 1\",\"sessionId\":null,\"startTime\":\"2019-07-16T11:21:20\",\"timeZoneId\":\"AUS Eastern Standard Time\",\"inputParameters\":\"{\\\"par1\\\":\\\"2000\\\",\\\"par2\\\":\\\"5000\\\"}\",\"logLevel\":1,\"isWindowless\":true,\"isEnabled\":true}"
headers = {'Content-Type': 'application/json',
'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Delete job schedule
DELETE /api/job/schedule/{scheduleId}
Deletes a specified job schedule.
scheduleId - The ID of the schedule to delete.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/schedule/b4cdc56a-2c16-4b1b-a135-b4e687f8bc95"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("DELETE", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Delete all job schedules
DELETE /api/job/{agentId}/schedules
Deletes all job schedules from a specified agent.
agentId - The ID of the agent.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/0041d691-49d1-4c48-8c21-654b8e248c12/schedules"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("DELETE", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Start job schedule
POST api/job/schedule/{scheduleId}/start
Starts a specified job schedule.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/schedule/d9c944df-93a7-ea77-f7b7-fa0b9c579917/start"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Disable job schedule
POST /api/job/schedule/{scheduleId}/off
Disables a specified job schedule.
scheduleId - The ID of the schedule to disable.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/schedule/3a8cc967-1158-e0ca-b1aa-d0f0eecd8d63/off"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Disable all job schedules
POST /api/job/{agentId}/schedules/off
Disables all job schedules for a specified agent.
agentId - The ID of the agent.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/0041d691-49d1-4c48-8c21-654b8e248c12/schedules/off"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Enable job schedule
POST /api/job/schedule/{scheduleId}/on
Enables a specified job schedule.
scheduleId - The ID of the schedule to enable.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/schedule/3a8cc967-1158-e0ca-b1aa-d0f0eecd8d63/on"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Enable all job schedules
POST /api/job/{agentId}schedules/on
Enables all job schedules for a specified agent
agentId - The ID of the agent.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/0041d691-49d1-4c48-8c21-654b8e248c12/schedules/on"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Set status for latest job run
POST api/job/{agentId}/status
Body: {"status": status}
Sets the specified status on the latest job run. The following job statuses are available:
0 - Never Run
1 - In Progress
2 - In Progress With Failure
3 - Succeeded
4 - Failure
5 - Failed
Example:
API Request
Python API RequestP
POST https://acc.sequentum.com/api/job/324046fc-375e-444d-87b7-ea2354c10932/status
Body: {"status": 5}
import requests
url = "https://acc.sequentum.com/api/job/324046fc-375e-444d-87b7-ea2354c10932/status"
payload = "{\"status\": 5}"
headers = {'Content-Type': 'application/json',
'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Get log for a specific agent run
GET api/run/{agentRunHistoryId}/log
Returns the log file for a specified agent run. The log file is compressed.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/run/9040f86d-bbc1-4038-9868-501145cc2370/log"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
with open("log.zip",'wb') as log:
log.write(response.content)
Get all logs for a specific job run
GET api/job/run/{jobRunHistoryId}/logs
Returns the log file for a specified agent run. The log file is compressed.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/run/6ef84ec7-d5e6-4a98-bbfa-0b67355a648f/logs"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
with open("logs.zip",'wb') as logs:
logs.write(response.content)
Get all data for a specific job run
GET api/job/run/{jobRunHistoryId}/backup
Returns data files for the specified job run. The data files are compressed. The agent or Sequentum server must be configured to store backup data.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/job/run/6ef84ec7-d5e6-4a98-bbfa-0b67355a648f/backup"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
with open("jobdata.zip",'wb') as jobdata:
jobdata.write(response.content)
Get data for a specific agent run
GET api/run/{agentRunHistoryId}/backup
Returns data files for a specified agent run. The data files are compressed. The agent or Sequentum server must be configured to store backup data.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/run/9040f86d-bbc1-4038-9868-501145cc2370/backup"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("GET", url, headers=headers, data = payload)
with open("rundata.zip",'wb') as rundata:
rundata.write(response.content)
Add or update agent input data
POST api/input/agent/{agentId}/csv?name={filename}
Body: Plain text CSV content.
Adds or updates input data for an agent. Input data will be updated if data with the same name already exists. Otherwise, the input data will be added to the agent.
agentId - The ID of the agent.
filename - The name of the input data. The extension of the file name should be .csv.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/input/agent/a2f22850-7c88-4e05-a30a-004aa980ee6c/csv?name=input.csv"
payload = "URL\r\nhttp://www.sequentum.com\r\nhttp://www.sequentum.com/help\r\nhttp://www.sequentum.com/account "
headers = {'Content-Type': 'application/json',
'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Upload agent input files
POST api/input/agent/{agentId}/files
Uploads one or more input files for an agent using multipart/form-data formatted file data.
Note: You can upload input files in either .csv or .txt or .zip format,where a zip file can have multiple csv or txt files.
Examples:
HTTP Request
CURL Request
.NET Request
Python Request
POST /api/input/agent/a2f22850-7c88-4e05-a30a-004aa980ee6c/files HTTP/1.1
Host: acc.sequentum.com
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ
----WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="filename"; filename="input2.csv.csv"
Content-Type: text/csv
curl -i -X POST -H "Content-Type: multipart/form-data" -H "Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6IlJvb3QiLCJTdGFtcCI6ImNjOWNiN2JhLWM0ZjItNGM0NS05ODY4LTMxM2Y5ZDRmZjhkZiIsIm5iZiI6MTU1ODQwNTI4NiwiZXhwIjoxNTkwMDI3NjgxLCJpc3MiOiJTZXF1ZW50dW0iLCJhdWQiOiJDb250ZW50IEdyYWJiZXIifQ.2pFQY9Ag8jndcL4jBSVLm_rhsZpmXXS8nn_crqXZ85c"
-F "data=@c:\test\input2.csv"
https://acc.sequentum.com/api/input/agent/a2f22850-7c88-4e05-a30a-004aa980ee6c/files
using (HttpClient httpClient = new HttpClient())
{
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6IlJvb3QiLCJTdGFtcCI6ImNjOWNiN2JhLWM0ZjItNGM0NS05ODY4LTMxM2Y5ZDRmZjhkZiIsIm5iZiI6MTU1ODQwNTI4NiwiZXhwIjoxNTkwMDI3NjgxLCJpc3MiOiJTZXF1ZW50dW0iLCJhdWQiOiJDb250ZW50IEdyYWJiZXIifQ.2pFQY9Ag8jndcL4jBSVLm_rhsZpmXXS8nn_crqXZ85c");
MultipartFormDataContent form = new MultipartFormDataContent();
byte[] fileBytes = File.ReadAllBytes(@"c:\test\input2.csv");
form.Add(new ByteArrayContent(fileBytes, 0, fileBytes.Length), "input2", "input2.csv");
HttpResponseMessage response = httpClient.PostAsync("https://acc.sequentum.com/api/input/agent/a2f22850-7c88-4e05-a30a-004aa980ee6c/files", form).Result;
}
import requests
url = " https://acc.sequentum.com/api/input/agent/a2f22850-7c88-4e05-a30a-004aa980ee6c/files"
payload = {}
files = [
('filename',open('/C:/test/input2.csv','rb'))
]
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload, files = files)
print(response.text.encode('utf8'))
Delete all agent input files
DELETE api/input/agent/{agentId}
Deletes all input files associated with the specified agent.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/input/agent/9040f86d-bbc1-4038-9868-501145cc2370"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("DELETE", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Disable All Agent Input Files
POST api/input/agent/{agentId}/disable
Disables all input files associated with the specified agent. Disabled input files are not deployed to the servers running the agent, so therefore not available to the agent as well.
Example:
API Request
Python API RequestP
import requests
url = "https://acc.sequentum.com/api/input/agent/9040f86d-bbc1-4038-9868-501145cc2370/disable"
payload = {}
headers = {'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6Imxva2VzaF9jaGFuZHJhIiwiU3RhbXAiOiI3N2Y0NWQ4NS0xMjU0LTRiNjctOGU2YS1iNzhhMWI1NDQ2YmMiLCJuYmYiOjE1NTg1MTgzNDIsImV4cCI6MTU5MDE0MDYyMiwiaXNzIjoiU2VxdWVudHVtIiwiYXVkIjoiQ29udGVudCBHcmFiYmVyIn0.0xU6_F_0sou5Q9Pco8iCR2JK8cVdtyqj_ER2sCyEdwQ'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Get SE Server Status
POST api/server/check
Body: {"key": "API key","ipAddress": "SE Server ipAddress","port":Port,"useSsl":true | false,"serverRefresh": true |false}
Gets the SE server status information.
key - API key added to access the SE server and can be found under the API key field on the Edit Server window on the Agent Control Center Servers page.
ipAddress- SE Server IP address.
port - Port to connect the SE server to Agent Control Center. The SE server is connected using the 8004 or 8005 port.
useSsl (optional) - Default value is False and is set to true when using SSL port 8005 to connect the SE server to ACC.
serverRefresh - Refreshes the SE server data and returns the current server status.
Example:
API Request
Python API RequestP
POST https://acc.sequentum.com/api/server/check
Body: {
"key": "3f8fb288098e4c749f18da856115f674",
"ipAddress": "127.0.0.1",
"port": 8004,
"useSsl": false,
"serverRefresh": true
}
import requests
import json
url ="https://acc.sequentum.com/api/server/check"
payload = json.dumps({
"key": "3f8fb288098e4c749f18da856115f674",
"ipAddress": "95.217.35.126",
"port": 8004,
"useSsl": False,
"serverRefresh": True
})
headers = {'Content-Type': 'application/json',
'Authorization': 'Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9uYW1laWRlbnRpZmllciI6IlJvb3QiLCJTdGFtcCI6ImNjOWNiN2JhLWM0ZjItNGM0NS05ODY4LTMxM2Y5ZDRmZjhkZiIsIm5iZiI6MTU1ODQwNTI4NiwiZXhwIjoxNTkwMDI3NjgxLCJpc3MiOiJTZXF1ZW50dW0iLCJhdWQiOiJDb250ZW50IEdyYWJiZXIifQ.2pFQY9Ag8jndcL4jBSVLm_rhsZpmXXS8nn_crqXZ85c'}
response = requests.request("POST", url, headers=headers, data = payload)
print(response.text.encode('utf8'))
Output:
{
"isError": false,
"message": "Connection to server 127.0.0.1:8004 is successful.",
"cpuUsages": "0%, a:2%, p:20%",
"memoryUsages": "6%, a:13%, p: 23 % ",
"discUsages": "C:47%",
"cpuThreshold": 100,
"memoryThreshold": 90
}
Postman
The Postman documentation provides a convenient way of testing API calls.
Click here to view the API Calls on Postman