Remote Storage File Name Transformation Script
The RemoteExportTransformation script can be used to transform the export file name and can also be used to modify the Compressed File names.
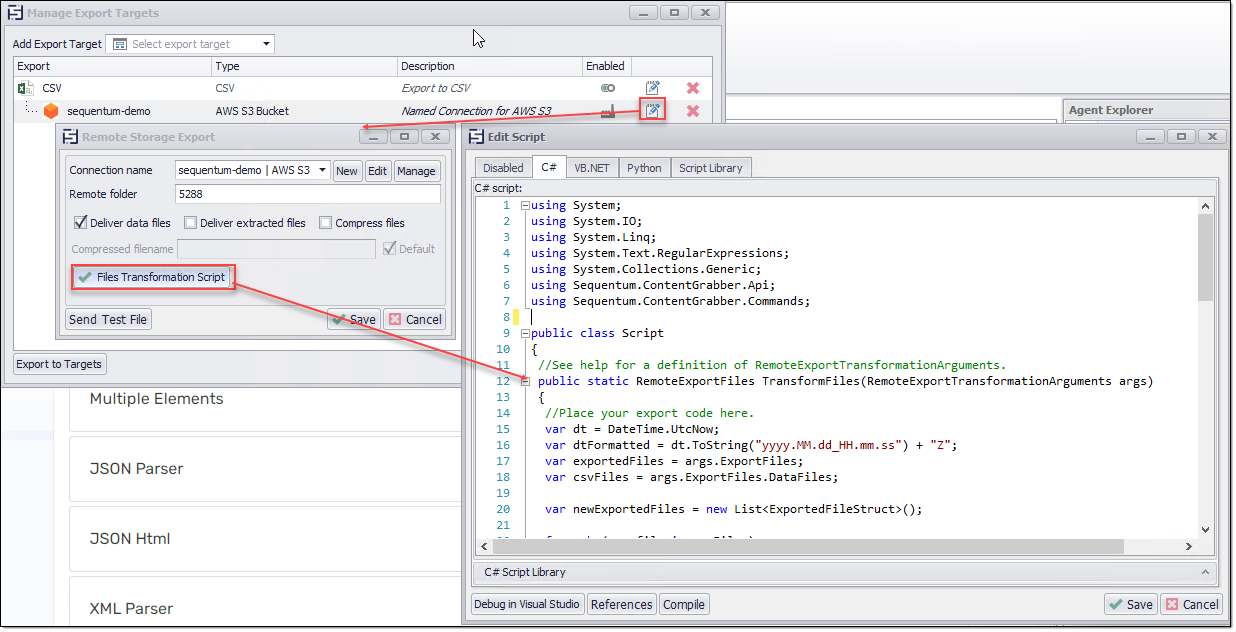
The following example creates different dynamic folders on the added remote storage based on the names of the different files and delivers the files in the respective folders:
NOTE:
To use the following code in the agent, use this additional library. By default, the library is not added in the RemoteExportTransformation Script.
using Sequentum.ContentGrabber.Commands;
using System.Linq;
using System.Text.RegularExpressions;
using System.Collections.Generic;
using System;
using System.IO;
using System.Linq;
using System.Text.RegularExpressions;
using System.Collections.Generic;
using Sequentum.ContentGrabber.Api;
using Sequentum.ContentGrabber.Commands;
public class Script
{
//See help for a definition of RemoteExportTransformationArguments.
public static RemoteExportFiles TransformFiles(RemoteExportTransformationArguments args)
{
//Place your export code here.
var dt = DateTime.UtcNow;
var dtFormatted = dt.ToString("yyyy.MM.dd_HH.mm.ss") + "Z";
var exportedFiles = args.ExportFiles;
var csvFiles = args.ExportFiles.DataFiles;
var newExportedFiles = new List<ExportedFileStruct>();
foreach (var file in csvFiles)
{
var filename = Path.GetFileName(file);
var filenameWithoutExt = Path.GetFileNameWithoutExtension(file);
newExportedFiles.Add(new ExportedFileStruct() { FilePath = file, TableName = filenameWithoutExt.ToLower()});
}
var remoteExportFiles = args.ExportFiles.ToRemoteExportFiles();
remoteExportFiles.UserFolderSeparator = true;
remoteExportFiles.FolderSeparator = ',';
args.ExportFiles.DataFiles = newExportedFiles.Select(x => {
var folder = Path.GetDirectoryName(x.FilePath);
var file = Path.GetFileName(x.FilePath);
return string.Format(@"{0}\{1}{2}{3}", folder, x.TableName.ToLower(), args.ExportFiles.ToRemoteExportFiles().FolderSeparator, file);
}).ToList();
remoteExportFiles.Clear();
remoteExportFiles.Assign(args.ExportFiles);
return remoteExportFiles;
}
struct ExportedFileStruct
{
public string FilePath;
public string TableName;
}
}